MPPG tutorial
Lets present an application which calculate body motion in gravitation field.
Simple mppg application
#include <mpp/mpp_window.h>
using namespace mpp;
int main(){
Mpp_window win;
return run();
}
Application with editable inputs
#include <mpp/mpp_window.h>
using namespace mpp;
double phi0=M_PI/4;
int main(){
Mpp_window win;
win.table.add_item("phi_0 = ", phi0);
return run();
}
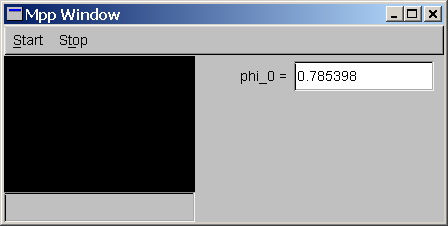
Lets draw something
#include <mpp/mpp_window.h>
#include <mpp/draw.h>
using namespace mpp;
double phi0=M_PI/4;
Scale scale(0.0, 1.0, 0.0, 1.0);
void ud(){
set_scale(scale);
set_color(FL_RED);
line(0,0,0.05*cos(phi0),0.05*sin(phi0));
}
int main(){
Mpp_window win;
win.table.add_item("phi_0 = ", phi0);
win.canvas.add_shape(new User_draw(ud));
return run();
}
Add calculation thread
#include <mpp/mpp_window.h>
#include <mpp/draw.h>
using namespace mpp;
double phi0=M_PI/4, v0=1.0;
double x=0.0, y=0.0, g=1.0;
double dt=1e-5;
Scale scale(0.0, 1.0, 0.0, 1.0);
void ud(){
set_scale(scale);
set_color(FL_RED);
line(0,0,0.05*cos(phi0),0.05*sin(phi0));
set_color(FL_YELLOW);
pointb(x,y);
}
void f(){
double vx=v0*cos(phi0), vy=v0*sin(phi0);
x=y=0.0;
while(may_i_repeat()){
vy+=-g*dt;
x+=vx*dt;
y+=vy*dt;
ask_update();
}
}
int main(){
Mpp_window win;
win.table.add_item("phi_0 = ", phi0);
win.table.add_item("dt = ", dt);
win.canvas.add_shape(new User_draw(ud));
set_work(f);
return run();
}
Add some shapes
#include <mpp/mpp_window.h>
#include <mpp/draw.h>
using namespace mpp;
double phi0=M_PI/4, v0=1.0;
double x=0.0, y=0.0, g=1.0;
double dt=1e-3;
Scale scale(0.0, 1.0, 0.0, 1.0,KEEP_RATIO);
Orbit orbit(10000,100,FL_RED, FL_YELLOW,scale);
Grid grid(0,0.2,0,0.2,scale);
void ud(){
set_scale(scale);
set_color(FL_RED);
line(0,0,0.05*cos(phi0),0.05*sin(phi0));
}
void f(){
double vx=v0*cos(phi0), vy=v0*sin(phi0);
x=y=0.0;
while(may_i_repeat()&&y>=0.0){
vy+=-g*dt;
x+=vx*dt;
y+=vy*dt;
orbit.add(x,y);
ask_update();
}
}
int main(){
Mpp_window win;
win.table.add_item("phi_0 = ", phi0);
win.table.add_item("dt = ", dt);
win.canvas.add_shape(new User_draw(ud));
win.canvas.add_shape(orbit);
win.canvas.add_shape(grid);
win.canvas.scale(scale);
set_work(f);
return run();
}